Object-Oriented Programming (OOP) – Basic Concepts
I published a new video lesson for beginners, where I explain the concepts of the object-oriented programming (OOP) with live code examples. The video is short, but is very informative and explains the OOP principles in a clear and easy to understand way, briefly, concisely, and in simple words. Learn about objects, classes, interfaces and inheritance in this short video with code examples in C# and JavaScript:
OOP Overview – Video Lesson
What Shall You Learn about OOP From This Lesson?
In this lesson I briefly explain and demonstrate the concept of OOP (the object-oriented programming), which is basically the concept of using classes and objects to model the real world in your programming language.
I explain the concepts of classes (which define the structure for the objects), data fields (which hold data) and properties (which provide access the data fields), and the concept of object state, as well as the actions, defined in the classes (which in OOP are called methods).
I show some live coding examples in C# and JavaScript where I define a class, instantiate objects from this class, and access the object’s data and call the methods of the object.
Later, I explain the concepts of inheritance, interfaces and abstract classes in OOP and provide a real-world example. I define an abstract class with an abstract method in it and inherit this base class into two child classes, where I override the inherited abstract methods to provide a specific implementation. Finally, I demonstrate how to use these abstract class and the child classes derived from it.
Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is the concept of using classes and objects to model the real world.
- Classes are sets of data fields, together with methods (which are functionality to interact with the data fields and other objects). Classes define the structure of information objects: the data they holds and the operation they can perform.
- Objects are instances of the classes, holding certain values in their data fields.
Let’s look at this simple example of class definition:
class Rectangle {
int width, height;
int CalcArea() {
return width * height;
}
}
The code above defines the class “Rectangle“, which holds two data fields: width and height (integer values). It defines a method, holding the code to calculate the area of the rectangle.
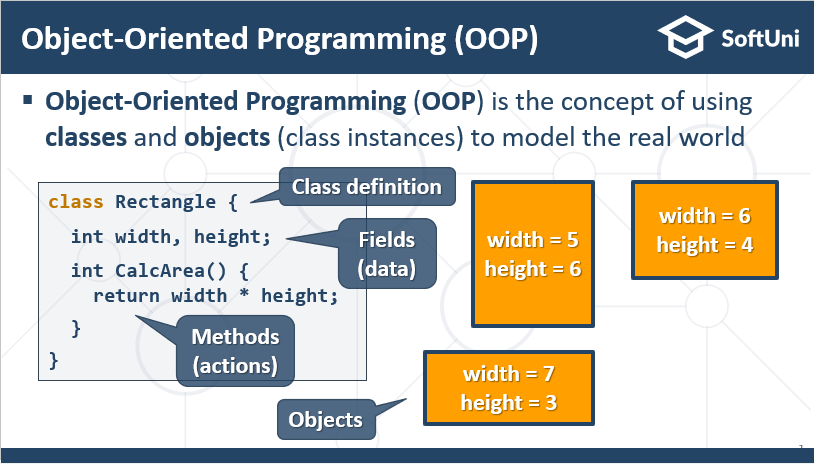
We can have several objects of this class “Rectangle“.
- The first object is a rectangle of width 5 and height 6.
- Another object has width 6 and height 4.
- Some other object has width 7 and height 3.
We have one class “Rectangle” and 3 objects (or instances) of this class.
- The class holds the definition (the specification, the model, the template) for the objects. It defines the data fields and methods and more details (in some cases).
- Classes don’t hold data. They hold data definitions and operation definitions.
- Objects hold values for the data fields in the class. Objects of class “Rectangle” hold data about certain rectangle. Objects are information structures, holding data.
- Typically, one class has multiple objects (or instances).
Classes and objects are the building blocks of the object-oriented programming (OOP) and they come together with some other OOP concepts like abstraction, interfaces, data encapsulation, inheritance, polymorphism and exception handling.
Examples of Classes and Objects in C# and JS
To demonstrate how classes and objects work in programming, I have prepared these examples in C# and JavaScript:
These examples demonstrate that in programming we can define classes, which model real-world entities. They hold data (properties) and operations, just like in the real world. Objects are instances of the class definition with certain data characteristics, just like objects in the real world.
Inheritance, Interfaces and Abstract Classes
Let’s continue with the concepts of inheritance, interfaces and abstract classes in the OOP, with a real-world example.
I can define an abstract class Figure with an abstract method calcArea() in it, and I can inherit this base class into two child classes (Circle and Rectangle), where I can override the inherited abstract method to provide a specific implementation for the area calculation. Finally, I can use this abstract class and the derived child classes as building block in my code.
Inheritance and interfaces are two other major concepts in the object-oriented programming.
- Inheritance allows classes to inherit data and functionality from a parent class (also called “base class”). When a class inherits another class, the parent class fields are merged with the child class fields and they together form the set of data fields for the child class.
- Interfaces defines abstract actions. These are actions to be implemented in the descendent classes. Interfaces define a set of empty (or abstract) methods (or actions), which shall be obligatory implemented in the child classes. Interfaces are also called “contracts“, because they define certain set of functionalities, a contract to implement certain methods.
Abstract and concrete classes are quite important when we model the real-world with the concepts from OOP.
- Abstract classes are used to model abstractions. For example, the class Figure is not a concrete figure like square or rectangle, but the concept or the abstraction of “figure”. Abstract classes defines data + actions (or normal methods) + abstract actions (or empty methods). Abstract classes are designed to be inherited (or extended).
- Concrete classes like Circle and Rectangle represent real entities, not abstractions. Concrete classes define data fields + concrete functionality (methods). They can implement interfaces and inherit abstract and other classes.
Inheritance and Interfaces – Example
In this example I demonstrate abstract classes and concrete classes. This is an example of abstract class, which models an abstraction “Figure“:
abstract class Figure {
int x, y;
abstract int calcArea();
}
The above code defines the base abstract class Rectangle with two data fields: x and y. It defines also an abstract action (or method) for calculating the area of the figure. This method is empty (or abstract), because it is specific to the concrete figure, like “circle” or “rectangle“. In the child (or descendent) classes this abstract action will become concrete, it will hold the code to calculate a circle area or rectangle area or other, depending on the concrete figure. This abstract class models the generic abstraction “Figure” and child classes will determine the type of the figure.
Please, don’t focus on the programming language. Focus on the OOP concepts now.
The abstract method calcArea() returns an integer value. It will be invoked by the child classes later.
This is an example of how we can define a child class “Circle”, which inherits from the abstract class “Figure”:
class Circle extends Figure {
int radius;
override int calcArea() =>
PI * radius * radius;
}
The above class inherits the fields “x” and “y” from “Figure” and appends an additional field “radius“. This way the child class has 3 fields: two inherited from the parent class and one defined additionally. The class Circle defines a concrete implementation of the abstract method calcArea(), which calculates the circle area using the well-known formula from the school-level math.
This is an example definition of another child class “Rectangle”, which inherits from the same base class Figure:
class Rectangle extends Figure {
int width, height;
override int calcArea() =>
width * height;
}
The “Rectangle” class defines two additional fields: width and height. It provides different concrete implementation of the calcArea() abstract method, which calculates the rectangle area.
This is a very good example of abstract and concrete classes. Abstract classes model common (or generic) data and functionality, and concrete classes model concrete entities and concrete implementations of the abstract actions from the parent class.
Note that the programming languages doesn’t matter for this examples. This configuration of parent class + child classes demonstrates an important concept from the object-oriented programming, which we shall learn in detail later. This example illustrates two main object-oriented programming concepts: inheritance and polymorphism.
Examples of Inheritance in OOP
To demonstrate how to use interfaces, abstract classes and inheritance in OOP, I have prepared these examples in C# and JavaScript:
Play with them to learn the concept of inheritance.
Subscribe to My YouTube Channel
Do you like this free programming lesson? Do you want more? Subscribe to my YouTube channel to stay in touch with my free coding courses, lessons and tutorials:
Join the “Learn to Code” Community
Join the SoftUni global learn-to-code community at softuni.org to get free access to the practical exercises and the automated judge system for this course. Get free help from mentors and meet other learners. It’s free!
Happy coding!
4 Responses to “Object-Oriented Programming (OOP) – Basic Concepts”
In what language is th8s?
This post is excellent. I am very happy to come to this post. Everything is explained very nicely. You have done a very well guide. thank you.
Than you for sharing this info. This is really helpful.
This information is so helpful for me!
scratch geometry dash